I originally posted this into an existing MD1200 thread:
Fun with an MD1200/MD1220 & SC200/SC220
This is a really good thread and kudos to @fohdeesha for being the first to post on interfacing with the MD1200 over the service port and reverse engineering some of the commands.
But I thought this deserved its own thread as it's become a bit of a project and would be better to not have it hidden on page 6.
(Would be nice to have an MD1200 master thread or wiki page to bring together the good work from everyone. There are a couple of other good threads with little gems sprinkled amongst them)
------
So I've successfully got an Arduino controlling the MD1200 and turning down the noisy fans.
Not exactly groundbreaking, and I'm sure I'm not the first to do this, but thought I'd put it here for others. Especially as a reminder to anyone who wants to play with it without buying the Dell password reset cable - the MD1200 uses RS232 12v line levels!!
When I first started trying to get this working without the Dell cable I had no luck. Then after sleeping on it I realised that the MD1200 is using RS232 line levels rather than TTL. I know @fohdeesha mentioned it was RS232 in their original post, but I'm a bit rusty on serial comms and forgot it uses +12v to -12v line levels and just went ahead trying to connect via standard TTL serial. Of course that didn't go well, and I ended up receiving garbage but clearly communicating something. So anyone else having a go at this on their own, make sure you remember this and you NEED a converter in the middle like a MAX232 to translate RS232 to TTL!
I actually had some MAX232 chips laying around, but decided to pop into Jaycar anyway and picked up a couple of RS-232 to TTL UART Converter Modules so I could avoid soldering until I had it working.
Anyway, I did up a little Arduino sketch which sets all the temps to 10 then sets the speed to 20. It works fine, so the next steps will be:
eg, I'd like for it to be able to poll for an uptime or something to see if the shelf has been restarted so I don't have to keep spoofing the temps over and over. I'd also like to periodically un-spoof the temps, read the real temps and spoof again. That way I could write extra logic to ramp up the fans in case it actually does get too hot. The danger in spoofing these temps and setting manual fan speeds is that it could end up getting too hot and fail, so it it would nice to have an extra safeguard. But it may end up causing the fans to momentarily ramp up and down during the temp read, which would get annoying, so it remains to be seen whether this is feasible or not.
BTW I've haven't had any success keeping the fans below 20%. I haven't tested it much, but it seems to still ramp back up even when the temps are spoofed. Anyone had any insights here?
Also, does anyone know a command that spits out uptime or something? I'd like to be able to detect when a shelf has rebooted and run that command frequently so I can minimise the time it spends on its full fan speed after reboot.
I'll upload the Arduino sketch and schematic once it's all working with both shelves and looping.
Here's the current (clumsy) code that just sends the commands once.
It might be a bit confusing because it's also processing serial commands from the onboard UART over USB which I used for testing. That one is "Serial". The port that communicates with the MD1200 is "remoteSerial" and is using the SoftwareSerial library to communicate over pins 7 and 8.
I'll probably keep the native serial code in there. That way I could still hook it up to my server via USB and send ad-hoc commands while still having the arduino sending the automated commands.
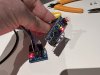
Fun with an MD1200/MD1220 & SC200/SC220
This is a really good thread and kudos to @fohdeesha for being the first to post on interfacing with the MD1200 over the service port and reverse engineering some of the commands.
But I thought this deserved its own thread as it's become a bit of a project and would be better to not have it hidden on page 6.
(Would be nice to have an MD1200 master thread or wiki page to bring together the good work from everyone. There are a couple of other good threads with little gems sprinkled amongst them)
------
So I've successfully got an Arduino controlling the MD1200 and turning down the noisy fans.
Not exactly groundbreaking, and I'm sure I'm not the first to do this, but thought I'd put it here for others. Especially as a reminder to anyone who wants to play with it without buying the Dell password reset cable - the MD1200 uses RS232 12v line levels!!
When I first started trying to get this working without the Dell cable I had no luck. Then after sleeping on it I realised that the MD1200 is using RS232 line levels rather than TTL. I know @fohdeesha mentioned it was RS232 in their original post, but I'm a bit rusty on serial comms and forgot it uses +12v to -12v line levels and just went ahead trying to connect via standard TTL serial. Of course that didn't go well, and I ended up receiving garbage but clearly communicating something. So anyone else having a go at this on their own, make sure you remember this and you NEED a converter in the middle like a MAX232 to translate RS232 to TTL!
I actually had some MAX232 chips laying around, but decided to pop into Jaycar anyway and picked up a couple of RS-232 to TTL UART Converter Modules so I could avoid soldering until I had it working.
Anyway, I did up a little Arduino sketch which sets all the temps to 10 then sets the speed to 20. It works fine, so the next steps will be:
- run those commands on a timed loop
- setup a second software serial port on two other pins so I can have the arduino controlling both shelves
- solder it all up properly and add the mini DIN 6 connectors
eg, I'd like for it to be able to poll for an uptime or something to see if the shelf has been restarted so I don't have to keep spoofing the temps over and over. I'd also like to periodically un-spoof the temps, read the real temps and spoof again. That way I could write extra logic to ramp up the fans in case it actually does get too hot. The danger in spoofing these temps and setting manual fan speeds is that it could end up getting too hot and fail, so it it would nice to have an extra safeguard. But it may end up causing the fans to momentarily ramp up and down during the temp read, which would get annoying, so it remains to be seen whether this is feasible or not.
BTW I've haven't had any success keeping the fans below 20%. I haven't tested it much, but it seems to still ramp back up even when the temps are spoofed. Anyone had any insights here?
Also, does anyone know a command that spits out uptime or something? I'd like to be able to detect when a shelf has rebooted and run that command frequently so I can minimise the time it spends on its full fan speed after reboot.
I'll upload the Arduino sketch and schematic once it's all working with both shelves and looping.
Here's the current (clumsy) code that just sends the commands once.
It might be a bit confusing because it's also processing serial commands from the onboard UART over USB which I used for testing. That one is "Serial". The port that communicates with the MD1200 is "remoteSerial" and is using the SoftwareSerial library to communicate over pins 7 and 8.
I'll probably keep the native serial code in there. That way I could still hook it up to my server via USB and send ad-hoc commands while still having the arduino sending the automated commands.
C:
#include <SoftwareSerial.h>
SoftwareSerial remoteSerial(7, 8); // RX, TX
byte incomingByte = 0;
byte outgoingByte = 0;
void setup() {
// Open serial communications and wait for port to open:
Serial.begin(38400);
while (!Serial) {
; // wait for serial port to connect. Needed for native USB port only
}
Serial.println("[LOCAL] Starting..");
// set the data rate for the SoftwareSerial port
pinMode(8, OUTPUT);
remoteSerial.begin(38400);
delay(500);
remoteSerial.write("\n\r");
delay(500);
remoteSerial.write("\n\r");
delay(500);
remoteSerial.write("set_temp 0 10\n\r\n\r");
delay(500);
remoteSerial.write("set_temp 1 10\n\r\n\r");
delay(500);
remoteSerial.write("set_temp 2 10\n\r\n\r");
delay(500);
remoteSerial.write("set_temp 3 10\n\r\n\r");
delay(500);
remoteSerial.write("set_temp 4 10\n\r\n\r");
delay(500);
remoteSerial.write("set_temp 5 10\n\r\n\r");
delay(500);
remoteSerial.write("set_speed 20\n\r");
}
void loop() { // run over and over
if (remoteSerial.available()) {
incomingByte = remoteSerial.read();
Serial.write(incomingByte);
}
if (Serial.available()) {
outgoingByte = Serial.read();
remoteSerial.write(outgoingByte);
}
}
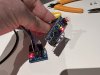
Last edited: